What is noods.io?
Noods.io is a great website for reading and storing recipes from the internet. Today, browsing the internet for recipes is very frustrating, needing to endlessly scroll past and avoid ads, cookies and prompts can make it difficult to find what you are looking for. This was ultimatelly the reason I decided to write my on basic online Cookbook: https://bignom.mpp.one/
Noods.io solves all the problems. If you find a good recipe just paste the url into noods and it will:
- import the recipe
- translate it to english
- add tags
- convert it into tickable ingredients
- convert it into tickable steps
- extract the approximate prepping and cooking time
- let you set the number of portions
Import script
To get all my recipes into noods.io i wrote a quick input script. It takes a text files as an input and imports the lines into noods.io.
Example input file (e.g. urls.txt):
https://www.gutekueche.at/rindercarpaccio-rezept-24595
https://bignom.mpp.one/rezept.php?id=55
https://bignom.mpp.one/rezept.php?id=56
https://bignom.mpp.one/rezept.php?id=57
Script:
#!/bin/bash
# Prompt for the Authorization token
read -p "Enter your Authorization token (Bearer format): " authToken
# Prompt for the text file containing the URLs
read -p "Enter the path to the text file containing the URLs: " filePath
# Check if the file exists
if [[ ! -f "$filePath" ]]; then
echo "Error: File not found at '$filePath'"
exit 1
fi
# Base API URL
apiBaseUrl="https://api.noods.io/recipeConversion"
# Read the file line by line
while IFS= read -r line; do
# Trim whitespace
url=$(echo "$line" | xargs)
# Skip empty lines
if [[ -z "$url" ]]; then
echo "Skipping empty line"
continue
fi
# Create the JSON payload
payload=$(jq -n --arg url "$url" '{url: $url}')
# Encode the payload
encodedPayload=$(jq -rn --argjson payload "$payload" '($payload|@uri)')
# Construct the full URL
fullUrl="$apiBaseUrl?input=$encodedPayload"
# Make the GET request
echo "Fetching data for URL: $url..."
response=$(curl -s -H "Authorization: $authToken" -H "Accept: text/event-stream" "$fullUrl")
# Print the response
echo "Response for URL $url:"
echo "$response"
echo "------------------------------------"
done < "$filePath"
To run the script you will need to install the package: jq
sudo apt install jq # For Debian/Ubuntu
sudo dnf install jq # For RHEL/Rocky/Fedora
brew install jq # For macOS
Make the script executable:
chmod +x fetch_recipes.sh
Run the script:
[user@host ~]$ ./fetch_recipes.sh
Enter your Authorization token (Bearer format): Bearer xxxx.xxx.xxxxxx...
Enter the path to the text file containing the URLs: urls.txt
Fetching data for URL: https://www.gutekueche.at/rindercarpaccio-rezept-24595...
Response for URL https://www.gutekueche.at/rindercarpaccio-rezept-24595:
data: {"type":"progress","progress":5}
[...]
data: {"type":"complete","data":{"url":"https://www.gutekueche.at/rindercarpaccio-rezept-24595","overview":{xxx}}
------------------------------------
Fetching data for URL: https://bignom.mpp.one/rezept.php?id=82...
Response for URL https://bignom.mpp.one/rezept.php?id=82:
data: {"type":"progress","progress":5}
[...]
data: {"type":"complete","data":{"url":"https://bignom.mpp.one/rezept.php?id=82","overview":{xxx}}
------------------------------------
First you need to paste in your Auth token. You can get that by opening the Network Tab in your local DEV-Tools in the Browser [F12]. Click on a request (e.g. cookbookRecipes?batch=1&input=%7B%7D), when you scroll down in your Header tab there will be a section called Requestheader. Inside there you will see a point Authorization and the Value starting with Baerer. Copy the whole block and paste it into the script when asked for it.
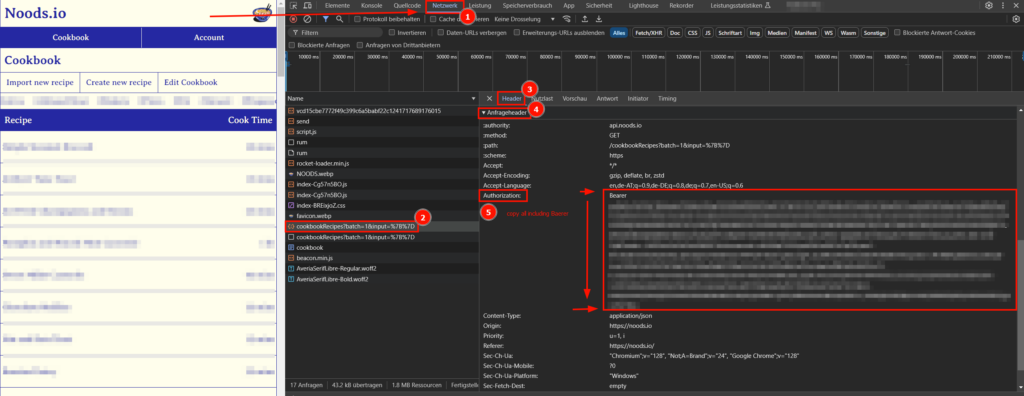
Your Token is only valid for a limited time, it will change once in a while.
Code on github: https://github.com/martpp/noods.io-import-script